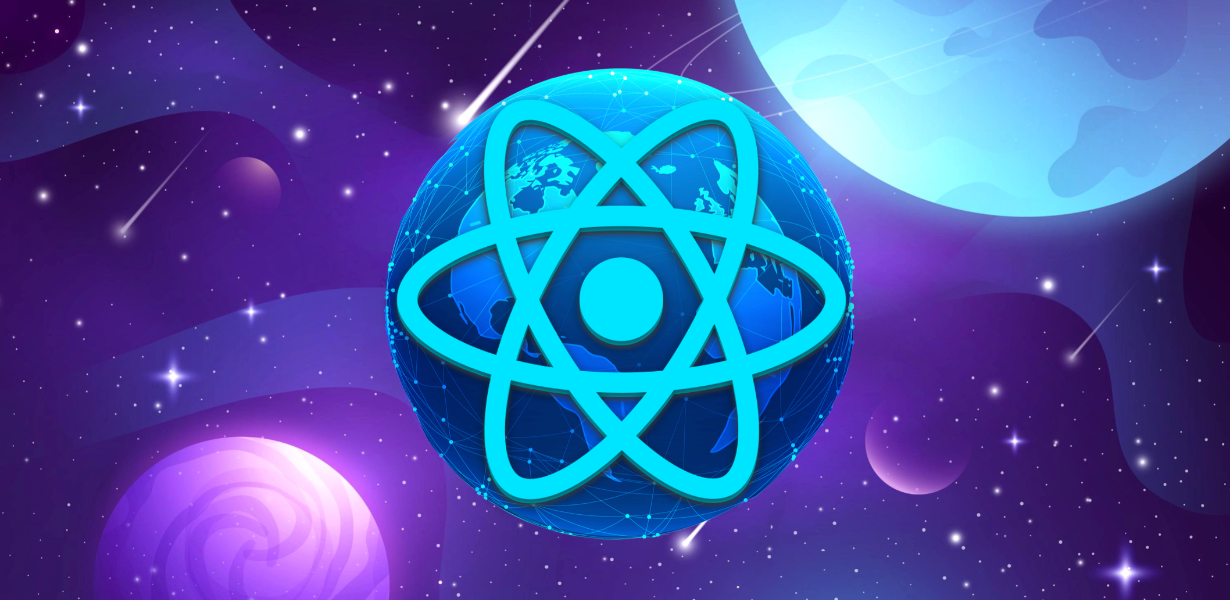
Mastering Reactive Forms in React.js: Advanced Techniques for Data Handling
- Post
- August 5, 2023
- JavaScript, React.js, Web Development
- 0 Comments
Reactive forms in React.js enable developers to build dynamic and interactive user interfaces. Unlike traditional HTML forms, reactive forms are built with a reactive programming approach, allowing components to respond to changes in data and user interactions in real-time. By harnessing the power of reactive forms, developers can create complex forms with validation, conditional logic, and custom behaviors, all while ensuring seamless data handling.
Understanding the Core Concepts
Before diving into advanced techniques, it’s essential to grasp the core concepts of reactive forms in React.js. Let’s explore the fundamental building blocks:
Form Controls
Form controls are the foundation of reactive forms. They represent individual input elements such as text fields, checkboxes, radio buttons, and select boxes. Each form control tracks its own value and validity, enabling developers to interact with and validate user input effortlessly.
Form Groups
Form groups help organize form controls. They allow developers to manage related form controls as a single unit, making it easier to apply validation and extract form data.
Form Validation
Validating user input is critical for maintaining data integrity. Reactive forms offer various validation mechanisms, such as required fields, minimum and maximum lengths, custom validators, and asynchronous validation.
Form Builder
The form builder is a powerful tool that simplifies the process of creating complex forms. It allows developers to define form structures programmatically, making it easy to add or remove form controls dynamically.
Reactive Form Events
Reactive forms provide a range of events that developers can leverage to respond to user interactions, form submissions, and validation status changes.
Advanced Techniques for Data Handling
Now that we have a solid understanding of the core concepts, let’s explore advanced techniques to master data handling in reactive forms:
Dynamic Form Controls
In some scenarios, you might need to create form controls dynamically based on user input or API responses. The form builder plays a key role here, allowing you to add and remove form controls on the fly.
Custom Validators
While the built-in validators are useful, you might encounter specific validation requirements unique to your application. By creating custom validators, you can tailor the validation process to suit your needs perfectly.
Nested Form Groups
Nested form groups enable you to build complex data structures and handle nested data efficiently. This technique is particularly useful when dealing with data objects containing nested properties.
Conditional Validation
There are instances where certain form controls should be validated based on the values of other form controls. Reactive forms provide elegant solutions to implement conditional validation rules effectively.
Asynchronous Validation
When validation depends on asynchronous operations, such as API calls or server-side validation, reactive forms offer seamless support for asynchronous validation.
Handling Form Submission
Handling form submissions and data processing is a critical aspect of reactive forms. Learn how to submit forms, manage form data, and handle form reset operations gracefully.
Form State Management
As forms grow in complexity, maintaining form state efficiently becomes crucial. Explore various state management techniques to ensure a smooth user experience.
Field Arrays
Field arrays allow developers to manage groups of form controls dynamically. This technique is valuable when dealing with repeated input fields or dynamic lists.
Form Layout and Design
A well-designed form enhances the user experience and improves usability. Learn how to structure and style your forms effectively for a polished look and feel.
Form Accessibility
Ensuring your forms are accessible to all users, including those with disabilities, is essential for creating inclusive applications. Discover techniques to make your reactive forms accessible.
Final Words
Mastering reactive forms in React.js empowers developers to build dynamic, interactive, and user-friendly applications with efficient data handling. By understanding the core concepts and exploring advanced techniques, you can take full advantage of reactive forms’ capabilities. Whether you are developing simple contact forms or complex data-driven applications, reactive forms will undoubtedly elevate your React.js projects to new heights.
Commonly Asked Questions
Q1: What is the difference between template-driven forms and reactive forms in React.js?
A1: Template-driven forms rely on two-way data binding and are easier to set up for simple use cases. On the other hand, reactive forms use a more robust reactive programming approach, offering greater control and flexibility for complex data handling scenarios.
Q2: Can I mix template-driven forms and reactive forms in the same React.js application?
A2: Yes, you can use both template-driven forms and reactive forms in the same application. However, it’s essential to be mindful of potential conflicts and choose the most appropriate approach for each form based on its complexity and requirements.
Q3: How can I handle asynchronous validation in reactive forms?
A3: Reactive forms provide the AsyncValidatorFn
function, allowing you to perform asynchronous validation operations such as API calls or server-side validation. You can use this function to validate form controls based on asynchronous responses.
Q4: Are there any third-party libraries that can enhance reactive forms in React.js?
A4: Yes, several third-party libraries can augment reactive forms’ capabilities, offering additional features and convenience. Some popular options include Formik, Redux-Form, and React-Hook-Form.
Q5: What is the recommended approach for handling form state in large-scale applications?
A5: In large-scale applications, managing form state can become complex. It is recommended to leverage state management libraries like Redux or MobX to handle form state globally and ensure better scalability and maintainability.